Frontend using AngularJS and TypeScript
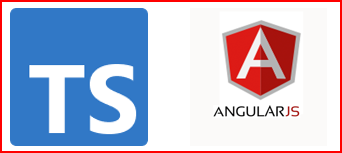
In this article, I will be developing a Model-View-Controller (MVC) application using AngularJS Framework, which would consume the REST APIs created in Microservices.
If you want to understand more about MVC Architecture, go through this article.
By the end of this article, you would learn about how to:
- Develop a Frontend Application using AngularJS Framework.
- How to call and consume REST APIs, from Application developed using AngularJS Framework.
If you have no time to read this article completely, but want to try the code for yourself, GitHub location is provided here.
Prerequisites
There are some prerequisites that are required for creating the Frontend Application.
Familiarity with Technologies and Frameworks
It is assumed that you have prior knowledge or familiarity with JavaScript, TypeScript and HTML. Because, I will not be covering the basics of these in this article.
If you are not familiar, then it is advised to get the basic knowledge of these and then come back to this site.
Node.js v8+
Download the latest version of the Node.js from here. Click the downloaded .msi and complete the installation.
Embedded npm available in the Node.js package.
webpack for building Frontend.
IDE for code development
You can use any IDE of your choice, that supports TypeScript. I will be using the Visual Studio Code.
If you wish to use the Visual Studio Code, download the latest version from here. Click on the downloaded .exe and complete the installation.
Create a Base AngularJS Project
The Angular CLI is used to create projects, generate application codes and library files, along with performing a variety of ongoing development tasks such as testing, bundling and deployment.
To install the Angular CLI, open a terminal window and run the following command:
npm install -g @angular/cli
To create a new workspace and initial starter app (where frontend-angular is the name of the project):
ng new frontend-angular
On some Windows 10 OS, running the above command would result in error message as shown below, because executing some scripts like ng are blocked, by default:
ng : File <npm_install_location>\npm\ng.ps1 cannot be loaded because running scripts is disabled on this system. For more information, see about_Execution_Policies at https://go.microsoft.com/fwlink/?LinkID=135170 . At line:1 char:1 + ng new frontend-angular + ~~ + CategoryInfo : SecurityError: (:) [], PSSecurityException + FullyQualifiedErrorId : UnauthorizedAccess |
Go to About Execution Policies and read the documentation on how to change the execution policy. Below commands can be used on the command prompt for changing the execution policy.
> Get-ExecutionPolicy -List
> Get-ExecutionPolicy -Scope CurrentUser
> Set-ExecutionPolicy -ExecutionPolicy RemoteSigned -Scope CurrentUser
After the execution policy is updated, you can run the ng new command again and this time it will be executed.
The command prompt provides you some information about features to include in the initial app. Accept the defaults by pressing the Enter or Return key.
The Angular CLI installs the necessary Angular npm packages and other dependencies. This can take a few minutes.
The initial startup project contains a simple vanilla Welcome app, ready to run.
Changing the port
By default, angular runs on port 4200
. If you want to change the port, there are two ways of doing it.
Through Command Line
Add the port
parameter when starting the application.
Navigate to the root of the workspace folder and run the following command:
ng serve --port 3000 -–open
Update the port in angular.json
file
- Open
angular.json
file and add a port option, as shown below (Only relevant properties are shown below):
{
"$schema": "./node_modules/@angular/cli/lib/config/schema.json",
"projects": {
"frontend-angular": {
"architect": {
"serve": {
"options": {
"port": 3000
}
}
}
}
}
}
Navigate to the root of the workspace folder and run the following command:
ng serve –-open
If your installation and setup was successful, you should see a page similar to the following, which would open automatically on your default browser: http://localhost:3000
The page you see is the Application Shell. The Shell is controlled by an Angular component named AppComponent.
The implementation of the shell AppComponent is distributed over three files:
app.component.ts
— the Component class code, written in TypeScript.app.component.html
— the Component Template, written in HTML.app.component.css
— the Component’s private CSS styles.
Adding Bootstrap Module to AngularJS Project
Bootstrap Module helps with some pre built CSS and JavaScript for styling and is used widely. In order to add the Bootstrap Module to our AngularJS project, follow the steps given below:
From inside the Project folder, type in the command:
npm install bootstrap
The Bootstrap’s latest versions assets will be installed in the node_modules/bootstrap
folder.
You can inform the AngularJS application where to look for Bootstrap, by adding the following line in the file src/styles.css
:
@import '~bootstrap/dist/css/bootstrap.min.css';
Enhancing the Vanilla Application to consume REST APIs
Let us start building the frontend components for our Todo Tracker Application, so that we can consume the REST APIs exposed by our microservice application, mentioned below:
Description | CRUD Operation | HTTP Method | REST API Endpoint |
---|---|---|---|
Create New Todo Task | CREATE | POST | /tasks |
Fetch All Todo Tasks | READ | GET | /tasks |
Fetch One Todo Task | READ | GET | /tasks/{id} |
Update One Specific Todo Task | UPDATE | PUT | /tasks |
Delete One Specific Todo Task | DELETE | DELETE | /tasks/{id} |
Display All items
Description | CRUD Operation | HTTP Method | REST API Endpoint |
---|---|---|---|
Fetch All Todo Tasks | READ | GET | /tasks |
For consuming this endpoint, we will need to add following components:
- Add New Components to the application by using the command
ng generate component todoApplication
The Angular CLI creates a new folder /src/app/todo-application/
and generates three files (like mentioned above) TodoApplicationComponent (.ts, .html, .css) along with a test file.
- Create a new TodoApplication Model =
todo-application.ts
1export interface TodoApplication {
2 id: number;
3 title: string;
4 description: string;
5 creationDate: Date;
6 dueDate: Date;
7 status: string;
8 todoTaskCommentsSet: any[];
9}
- Create a new TodoTaskComments Model =
to-do-task-comments.ts
1export interface TodoTaskComments {
2 todoTaskCommentsId: number;
3 taskComments: string;
4 creationDate: Date;
5}
Hardcode the values
- Update the TodoApplication Component Class =
todo-application.component.ts
1import { Component, OnInit } from '@angular/core';
2import {TodoApplicaton } from './todo-application';
3import { TodoTaskComments } from "./to-do-task-comments";
4
5@Component({
6 selector: 'app-todo-application',
7 templateUrl: './todo-application.component.html',
8 styleUrls: ['./todo-application.component.css']
9})
10export class TodoApplicationComponent implements OnInit {
11 todoApplication:TodoApplication;
12 todoTaskComments: TodoTaskComments;
13
14 constructor() { }
15
16 ngOnInit() {
17 this.retrieveAllTodoList();
18 }
19
20 retrieveAllTodoList() {
21 this.todoTaskComments = {
22 todoTaskCommentsId:2,
23 taskComments:'Comment Hardcoded in Angular',
24 creationDate: new Date()
25 };
26
27 var todoTaskCommentsArray= [];
28 todoTaskCommentsArray.push(this.todoTaskComments);
29
30 var data: any[];
31 data = [{
32 id:1,
33 title:'Hardcoded in Angular ',
34 description:'Data is hardcoded in Angular',
35 creationDate: new Date(),
36 dueDate: new Date(),
37 status:'NOT_STARTED',
38 todoTaskCommentsSet:todoTaskCommentsArray
39 }];
40
41 this.todoApplication = data;
42 }
43}
Some explanation about highlighted lines:
-
Line# 4-8: Since this is a Component Class, this is annotated with
@Component
.- selector: This is the tag used on the parent component to identify this Component.
- templateUrl: This is the HTML template for this Component.
- styleUrls: This is the private css of this Component. Styles written in this file would override all global styles for this Component.
-
Line# 20-41: Hardcoded values for the TodoApplication and TodoTaskComments models.
- Update the TodoApplication Component Template =
todo-application.component.html
1<div className="container">
2 <div className="container">
3 <table class='table-striped' border='1' align="center">
4 <thead>
5 <tr>
6 <th>Title</th>
7 <th>Description</th>
8 <th>Due Date</th>
9 <th>Status</th>
10 <th>No. Of Comments</th>
11 </tr>
12 </thead>
13 <tbody>
14 <tr *ngFor="let todoApp of todoApplication">
15 <td> {{todoApp.title}} </td>
16 <td> {{todoApp.description}} </td>
17 <td> {{todoApp.dueDate | date: 'dd-MMM-yyyy' }} </td>
18 <td> {{todoApp.status}} </td>
19 <td> {{todoApp.todoTaskCommentsSet.length}} </td>
20 </tr>
21 </tbody>
22 </table>
23 </div>
24</div>
- To view the TodoApplication Component, update the App Component template =
app.component.html
1<div style="text-align: center;">
2 <h1>{{title}}</h1>
3 <app-todo-application></app-todo-application>
4</div>
- Line# 3: This is the selector attribute of the Component, explained above.
When the browser refreshes, if your updates are fine, you should see page similar to the following.
At this stage, we are able to display the list of components that are hardcoded onto our Angular Component.
Connect REST API with the Angular Project
Before we continue with connecting our Angular to REST API, we need to add modules required to make the REST API calls – HttpClient
- Add New Service Component to the application by using the command:
ng generate service todoService
The Angular CLI generates two files todo-service.service (.ts, .spec.ts) in folder /src/app
.
- Update the Service Component =
todo-service.service.ts
1import { Injectable } from '@angular/core';
2import { HttpClient } from '@angular/common/http';
3
4@Injectable({
5 providedIn: 'root'
6})
7export class TodoServiceService {
8
9 private TODO_API_URL = 'http://localhost:8080/todo-app/tasks';
10
11 constructor(private httpClient: HttpClient) { }
12
13 public retrieveAllTodoList(){
14 return this.httpClient.get(this.TODO_API_URL);
15 }
16}
- Update the TodoApplication Component Class =
todo-application.component.ts
1import { TodoServiceService } from '../todo-service.service';
2
3export class TodoApplicationComponent implements OnInit {
4 constructor(private todoService: TodoServiceService) { }
5
6 retrieveAllTodoList() {
7 this.todoService.retrieveAllTodoList().subscribe((data: TodoApplication) => {
8 console.log(data);
9 })
10 }
11}
At this moment, when the browser refreshes, we get following error in console.
1core.js:4610 ERROR NullInjectorError: R3InjectorError(AppModule)
2 [TodoServiceService -> HttpClient -> HttpClient -> HttpClient]:
3 NullInjectorError: No provider for HttpClient!
4 at NullInjector.get (http://localhost:4200/vendor.js:11932:27)
5 at R3Injector.get (http://localhost:4200/vendor.js:22439:33)
6 at R3Injector.get (http://localhost:4200/vendor.js:22439:33)
7 at R3Injector.get (http://localhost:4200/vendor.js:22439:33)
8 at injectInjectorOnly (http://localhost:4200/vendor.js:11818:33)
9 at Module.ɵɵinject (http://localhost:4200/vendor.js:11822:57)
10 at Object.TodoServiceService_Factory [as factory] (http://localhost:4200/main.js:191:159)
11 at R3Injector.hydrate (http://localhost:4200/vendor.js:22607:35)
12 at R3Injector.get (http://localhost:4200/vendor.js:22428:33)
13 at NgModuleRef$1.get (http://localhost:4200/vendor.js:35872:33)
To remove this error, we need to add the HttpClientModule in the app.module.ts
1import { BrowserModule } from '@angular/platform-browser';
2import { NgModule } from '@angular/core';
3import { HttpClientModule } from '@angular/common/http';
4
5import { AppComponent } from './app.component';
6import { TodoApplicationComponent } from './todo-application/todo-application.component';
7
8@NgModule({
9 declarations: [
10 AppComponent,
11 TodoApplicationComponent
12 ],
13 imports: [
14 BrowserModule,
15 HttpClientModule
16 ],
17 providers: [],
18 bootstrap: [AppComponent]
19})
20export class AppModule { }
When we refresh the browser, we get another error, this time CORS Request Error.
Access to XMLHttpRequest at 'http://localhost:8080/todo-app/tasks' from origin 'http://localhost:4200'
has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
The microservice application’s REST API is running on http://localhost:8080/
, and it is not allowing requests from other servers/domains - http://localhost:4200/
(in our case here).
This is called CORS (Cross-Origin Resource Sharing) policy, where in by default, servers block request coming from other servers or domains.
Click here to know more about how to enable CORS Policy and resolve this error.
After enabling CORS on Microservice Application, if we refresh the browser - http://localhost:4200/
, we should see the output from REST APIs microservice application, printed on the browser console (Click Developer Tools from settings or F12 on most browsers).
Display REST API results on Angular Module
- Update the TodoApplication Component Class =
todo-application.component.ts
1export class TodoApplicationComponent implements OnInit {
2 retrieveAllTodoList() {
3 this.todoService.retrieveAllTodoList().subscribe((data: TodoApplication) => {
4 this.todoApplication = data;
5 })
6 }
7}
- Line# 4: Replace the console output with the object to be displayed on UI.
When the browser refreshes, if your updates are fine, you should see page similar to the following, with result coming from Microservice project’s REST APIs.
Congratulations, you have successfully integrated your Angular application with the REST API
Display One Item
To display only one item, we need to update the following components.
- Update the TodoApplication Component Class =
todo-application.component.ts
1export class TodoApplicationComponent implements OnInit {
2 todoApplication:TodoApplication;
3 isSinglePageView: Boolean = false;
4
5 constructor(private todoService: TodoServiceService) { }
6
7 view(event, todoApp) {
8 this.todoApplication = todoApp;
9 this.isSinglePageView = true;
10 }
11}
- Update the TodoApplication Component Template =
todo-application.component.html
, to include the VIEW button and a section for the single item view to be displayed when we click on VIEW button.
1<div className="container">
2 <div class="container" *ngIf="!isSinglePageView">
3 <table className='table-striped' border='1' align="center">
4 <thead>
5 <tr>
6 <th>Title</th>
7 <th>Description</th>
8 <th>Due Date</th>
9 <th>Status</th>
10 <th>No. Of Comments</th>
11 <th>View</th>
12 </tr>
13 </thead>
14 <tbody>
15 <tr *ngFor="let todoApp of todoApplication">
16 <td> {{todoApp.title}} </td>
17 <td> {{todoApp.description}} </td>
18 <td> {{todoApp.dueDate | date: 'dd-MMM-yyyy' }} </td>
19 <td> {{todoApp.status}} </td>
20 <td>{{todoApp.todoTaskCommentsSet.length}}</td>
21 <td><button class="btn btn-success" (click)="view($event, todoApp)">VIEW</button></td>
22 </tr>
23 </tbody>
24 </table>
25 </div>
26 <div *ngIf="isSinglePageView">
27 <table class='table-striped' border='1' align="center">
28 <tbody>
29 <tr>
30 <th>Title</th>
31 <td> {{todoApplication.title}} </td>
32 </tr><tr>
33 <th>Description</th>
34 <td> {{todoApplication.description}} </td>
35 </tr><tr>
36 <th>Creation Date</th>
37 <td> {{todoApplication.creationDate | date: 'dd-MMM-yyyy' }} </td>
38 </tr><tr>
39 <th>Due Date</th>
40 <td> {{todoApplication.dueDate | date: 'dd-MMM-yyyy' }} </td>
41 </tr><tr>
42 <th>Status</th>
43 <td> {{todoApplication.status}} </td>
44 </tr><tr>
45 <th>
46 Comments
47 </th>
48 <td>
49 <table class='table-striped' border='1' align="center"
50 *ngIf="todoApplication.todoTaskCommentsSet.length > 0">
51 <thead>
52 <tr>
53 <th>Creation Date</th>
54 <th>Description</th>
55 </tr>
56 </thead>
57 <tbody>
58 <tr *ngFor="let todoComments of todoApplication.todoTaskCommentsSet">
59 <td>{{todoComments.creationDate | date: 'dd-MMM-yyyy' }}</td>
60 <td> {{todoComments.taskComments}} </td>
61 </tr>
62 </tbody>
63 </table>
64 </td>
65 </tr>
66 </tbody>
67 </table>
68 </div>
69</div>
When the browser refreshes, if your updates are fine, you should see page similar to the following:
On clicking VIEW button
Update One Item
Description | CRUD Operation | HTTP Method | REST API Endpoint |
---|---|---|---|
Update One Specific Todo Task | UPDATE | PUT | /tasks |
- Update the TodoService Service Component =
todo-service.service.ts
1export class TodoServiceService {
2
3 private TODO_API_URL = 'http://localhost:8080/todo-app/tasks';
4
5 constructor(private httpClient: HttpClient) { }
6
7 public updateItem(todoApp: TodoApplication){
8 return this.httpClient.put(this.TODO_API_URL, todoApp);
9 }
10
11 public retrieveStatus(){
12 return this.httpClient.get(this.TODO_API_URL+'/status');
13 }
14}
- Line# 7-9: Call the REST API with HTTP Method - PUT.
- Line# 11-13: Call the REST API for getting the status options.
- Update the TodoApplication Component Class =
todo-application.component.ts
1export class TodoApplicationComponent implements OnInit {
2 todoApplication:TodoApplication;
3 isSinglePageView: Boolean = false;
4 todoTaskComments: TodoTaskComments;
5 isEdit: Boolean = false;
6 todoStatus = [];
7 showToggleCommentTable:boolean = false;
8
9 constructor(private todoService: TodoServiceService) { }
10
11 ngOnInit() {
12 this.retrieveAllTodoList();
13 this.retrieveStatus();
14 }
15
16 edit(event, todoApp:TodoApplication) {
17 this.todoApplication = todoApp;
18 this.todoTaskComments = {todoTaskCommentsId:null, taskComments:'', creationDate: null};
19 this.isEdit = true;
20 this.isSinglePageView = true;
21 }
22
23 submit(todoApplication:TodoApplication, todoTaskComments:TodoTaskComments) {
24 if(todoApplication.systemTasksId != null) {// For Update
25 var todoTaskCommentsArray= [];
26 todoTaskCommentsArray.push(todoTaskComments);
27 todoApplication.todoTaskCommentsSet=todoTaskCommentsArray;
28
29 this.todoService.updateItem(todoApplication).subscribe((data: TodoApplication) => {
30 this.todoApplication = data;
31 })
32 }
33 location.reload();
34 }
35
36 retrieveStatus() {
37 this.todoService.retrieveStatus().subscribe((data: any[]) => {
38 this.todoStatus = data;
39 })
40 }
41
42 toggleCommentsTable(event){
43 this.showToggleCommentTable = !this.showToggleCommentTable;
44 }
45}
- Line# 16-21: When the edit button is clicked on the html component, edit method is invoked.
- Line# 23-34: When the submit button is clicked on the html component, submit method is invoked and
updateItem
method from Service is called. - Line# 42-44: Show/Hide the “Add New Comments” section based on button click.
- Update the TodoApplication Component Template =
todo-application.component.html
- To add the
Edit Button
to the Listing Page.
- To add the
1<div class="container">
2 <!-- Start of div for displaying the list -->
3 <div class="container" *ngIf="!isSinglePageView">
4 <table class='table-striped' border='1' align="center">
5 <thead>
6 <tr>
7 <th>Title</th>
8 <th>Description</th>
9 <th>Due Date</th>
10 <th>Status</th>
11 <th>No. Of Comments</th>
12 <th>View</th>
13 <th>Edit</th>
14 </tr>
15 </thead>
16 <tbody>
17 <tr *ngFor="let todoApp of todoApplication">
18 <td> {{todoApp.title}} </td>
19 <td> {{todoApp.description}} </td>
20 <td> {{todoApp.dueDate | date: 'dd-MMM-yyyy' }} </td>
21 <td> {{todoApp.status}} </td>
22 <td>{{todoApp.todoTaskCommentsSet.length}}</td>
23 <td><button class="btn btn-success" (click)="view($event, todoApp)">VIEW</button></td>
24 <td><button class="btn btn-success" (click)="edit($event, todoApp)">EDIT</button></td>
25 </tr>
26 </tbody>
27 </table>
28 </div>
29 <!-- End of div for displaying the list -->
30</div>
- To update the Single View Page for Editing
- When
isEdit
is False, View Only - When
isEdit
is True, allow fields to be Edited.
- When
[(ngModel)]
is the Angular style of Two Way Data Binding
1<div class="container">
2
3 <!-- Start of div for displaying the single item -->
4 <div *ngIf="isSinglePageView">
5 <table class='table-striped' border='1' align="center">
6 <tbody>
7 <tr>
8 <th>Title</th>
9 <td *ngIf="!isEdit"> {{todoApplication.title}} </td>
10 <td *ngIf="isEdit"><input [(ngModel)]="todoApplication.title" size="35" /></td>
11 </tr><tr>
12 <th>Description</th>
13 <td *ngIf="!isEdit">{{todoApplication.description}}</td>
14 <td *ngIf="isEdit"><textarea [(ngModel)]="todoApplication.description" rows="3" cols="38"></textarea></td>
15 </tr><tr>
16 <th>Creation Date</th>
17 <td> {{todoApplication.creationDate | date: 'dd-MMM-yyyy' }} </td>
18 </tr><tr>
19 <th>Due Date</th>
20 <td *ngIf="!isEdit"> {{todoApplication.dueDate | date: 'dd-MMM-yyyy' }} </td>
21 <td *ngIf="isEdit"> <input type="date" [(ngModel)]="todoApplication.dueDate" /> </td>
22 </tr><tr>
23 <th>Status</th>
24 <td *ngIf="!isEdit"> {{todoApplication.status}} </td>
25 <td *ngIf="isEdit">
26 <select [(ngModel)]="todoApplication.status">
27 <option value="">--Select Status--</option>
28 <option *ngFor="let status of todoStatus" [ngValue]="status">{{status}}</option>
29 </select>
30 </td>
31 </tr><tr *ngIf="todoApplication.systemTasksId != null">
32 <th>
33 Comments
34 </th>
35 <td>
36 <table class='table-striped' border='1' align="center"
37 *ngIf="isEdit || todoApplication.todoTaskCommentsSet.length > 0">
38 <thead>
39 <tr>
40 <th>Creation Date</th>
41 <th>Description</th>
42 </tr>
43 </thead>
44 <tbody>
45 <tr *ngFor="let todoComments of todoApplication.todoTaskCommentsSet">
46 <td>{{todoComments.creationDate | date: 'dd-MMM-yyyy' }}</td>
47 <td> {{todoComments.taskComments}} </td>
48 </tr>
49 <tr *ngIf="isEdit">
50 <td colspan="2">
51 <button class="btn btn-success" (click)="toggleCommentsTable()">Add New Comments</button>
52 </td>
53 </tr>
54 <tr>
55 <td colspan="2">
56 <table *ngIf="showToggleCommentTable">
57 <tr>
58 <th>Description</th>
59 <td>
60 <textarea [(ngModel)]="todoTaskComments.taskComments" rows="2" cols="24"></textarea>
61 </td>
62 </tr>
63 </table>
64 </td>
65 </tr>
66 </tbody>
67 </table>
68 </td>
69 </tr><tr *ngIf="isEdit">
70 <td colspan="2"><button class="btn btn-success"
71 (click)="submit(todoApplication, todoTaskComments)">SUBMIT</button></td>
72 </tr>
73 </tbody>
74 </table>
75 </div>
76 <!-- End of div for displaying the single item -->
77</div>
When the browser refreshes, if your updates are fine, you should see page similar to the following.
On clicking EDIT button
On clicking ADD NEW COMMENTS button
On clicking SUBMIT button, after updates
Delete one item from the list
Description | CRUD Operation | HTTP Method | REST API Endpoint |
---|---|---|---|
Delete One Specific Todo Task | DELETE | DELETE | /tasks/{id} |
- Update the TodoService Service Component =
todo-service.service.ts
1export class TodoServiceService {
2
3 private TODO_API_URL = 'http://localhost:8080/todo-app/tasks';
4
5 constructor(private httpClient: HttpClient) { }
6
7 public deleteItem(id:number){
8 return this.httpClient.delete(this.TODO_API_URL+'/'+id);
9 }
10}
- Update the TodoApplication Component Class =
todo-application.component.ts
1export class TodoApplicationComponent implements OnInit {
2 delete(todoApp:TodoApplication) {
3 this.todoService.deleteItem(todoApp.systemTasksId).subscribe((data: string) => {})
4 location.reload();
5 }
6}
- Update the TodoApplication Component Template =
todo-application.component.html
1<div className="container">
2 <div className="container" *ngIf="!todoApp">
3 <table className='table-striped' border='1' align="center">
4 <thead>
5 <tr>
6 <th>Delete</th>
7 </tr>
8 </thead>
9 <tbody>
10 <tr *ngFor="let todoApp of todoApplication">
11 <td><button class="btn btn-warning" (click)="delete($event, todoApp)">DELETE</button></td>
12 </tr>
13 </tbody>
14 </table>
15 </div>
16</div>
When the browser refreshes, if your updates are fine, you should see page similar to the following.
On clicking DELETE button
Create New Item
Description | CRUD Operation | HTTP Method | REST API Endpoint |
---|---|---|---|
Create New Todo Task | CREATE | POST | /tasks |
- Update the TodoService Component Class =
todo-service.service.ts
1export class TodoServiceService {
2
3 private TODO_API_URL = 'http://localhost:8080/todo-app/tasks';
4
5 constructor(private httpClient: HttpClient) { }
6
7 public createItem(todoApp: TodoApplication){
8 return this.httpClient.post(this.TODO_API_URL, todoApp);
9 }
10}
- Update the TodoApplication Component Class =
todo-application.component.ts
1export class TodoApplicationComponent implements OnInit {
2
3 create() {
4 this.todoApplication = {systemTasksId:null, title:'', description:'', creationDate: null, dueDate: new Date(),
5 status:'', todoTaskCommentsSet:null};
6 this.isEdit = true;
7 this.isSinglePageView = true;
8 }
9
10 submit(todoApplication:TodoApplication, todoTaskComments:TodoTaskComments) {
11 if(todoApplication.systemTasksId != 0) {// For Update
12 var todoTaskCommentsArray= [];
13 todoTaskCommentsArray.push(todoTaskComments);
14 todoApplication.todoTaskCommentsSet=todoTaskCommentsArray;
15
16 this.todoService.updateItem(todoApplication).subscribe((data: TodoApplication) => {
17 this.todoApplication = data;
18 })
19 }
20 else { //For Create, id = 0
21 this.todoService.createItem(todoApplication).subscribe((data: TodoApplication) => {
22 this.todoApplication = data;
23 })
24 }
25 location.reload();
26 }
27}
- Update the TodoApplication Component Template =
todo-application.component.html
1<div className="container">
2 <div class="container" *ngIf="!isSinglePageView">
3 <button class="btn btn-success" (click)="create()">CREATE</button>
4 </div>
5</div>
When the browser refreshes, if your updates are fine, you should see page similar to the following.
On clicking CREATE button
After submission
Conclusion
With this setup complete, we have come to the end of this article.
At the end of this article, we have learned how to:
- Develop a Frontend Application using AngularJS Framework.
- How to call and consume REST APIs, from Application developed using AngularJS Framework.
Complete code for this project can be found at GitHub here. Go ahead and clone it.
Instructions on how to clone and run the project are provided on the GitHub page.